So your teacher said to you, “Make me a website that saves some data to the cloud.” And instead of teaching you, they forced you to find the answers for yourself. So you’ve been crawling around the internet — scraping your hands and knees on the broken glass of PHP tutorials and garbage.
Take a deep breath and relax. Papa David is here for you. It’s all good now. Just keep reading and copying the code at the end.
Many years ago, I created my first web program. I wanted to make a program that would help with math, similar to “Wolfram-alpha.” At that time, I didn’t know any good design practices; I had a lot of JavaScript files, and the import order in the HTML file was important. And the dependencies between the files were implicit. It was bad times.
I know what it’s like to be a noob.
But since then, I’ve worked at a couple of companies and done a couple of personal projects. So I learned better practices. I’m going to share something basic and just hint at tips for improvement because the main goal here is to finish your homework.
I have no idea what you are creating specifically, so allow me to provide an example.
What are we creating?
I’m reading the “Game of Thrones.” It’s a big book. And I want a program to keep my page, like a virtual bookmark. It will start at the number zero, and as I read I can change the number. If I reload the page, it’ll still remember my number.
Step-by-step instructions
Introduction
What is an internet application? Typically, it’s a website that connects to a server for data and then displays it to the user. The part that the user sees is called the frontend. And the other part is called the backend.
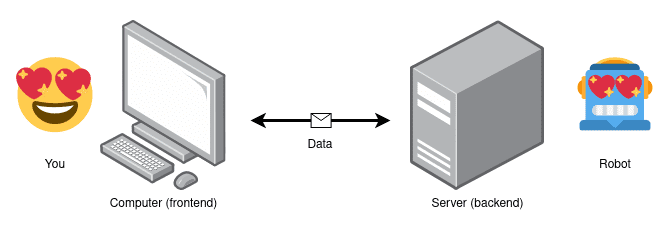
When a person says “endpoint,” they mean a URL and a verb. Verbs like get, create, update, and delete. If you don’t understand it, skip it for now, I’ll explain later.
When a person says “JSON,” they don’t mean the name, but it’s a format for data, similar to CSV or TXT.
That’s all you should understand.
Choose technologies
With web development there are many decisions you can make, especially at the beginning. It’s overwhelming. I recommend keeping stuff simple, sounds good?
If you have prior knowledge of a programming language, I recommend that. But since we need a user interface, we’re going to need JavaScript and HTML, there’s no escaping it. So I’ll be using JavaScript as a frontend language and also as a backend language via Nodejs.
Obtain data
When making a feature (or a project) there is a common question where to start. It’s a matter of preference; and since you are with me, we will do it my way. I like to start with what I think of as the center and expand outwards.
I envision the database as a dot, with the backend wrapping around it. And then the frontend around that. But I don’t like to start with the database because it feels like an implementation detail. If you don’t understand, it’s okay.
And our example has two use cases, reading the number and updating the number. It’s easier to start reading. Because when we start updating, it’s hard to verify that it’s functional. Ya know?
Backend
Let’s start with the backend; with Nodejs the servers can be super simple.
- Install Nodejs.
- Make a new folder for the project.
- Inside the folder, run
npm init --yes
from the command line. Using “yes” will skip all questions. - Run
npm install express --save
, this will install a dependency we are about to use. - Copy the code below into a file called “server.js” please.
const express = require('express')
const app = express()
app.get('/bookmark', (req, res) => {
res.json({ page: 0 })
})
app.listen(8080, () => {
console.log('Server is running on http://localhost:8080')
})
- You can start it by typing
node ./server.js
in the command line. - The server is great; it’s easy. Go to http://localhost:8080/bookmark
- Boom! 🎉 You have a working backend. 😋 Do some squats bro! Of course it’s not the final version, calm down.
Frontend
The frontend is the front. But now is the time to explain the endpoint verbs. Buckle your seat belt. When you’re roaming the internet, you’re mostly making GET requests, which are requests to see stuff. When you submit stuff, it’s probably a POST request.
For now, we’re just going to use the GET endpoint we already have. Delicious.
- Create a new file called “home.html” in the same folder.
- Copy the code below:
<html>
<head>
<script>
const xhr = new XMLHttpRequest()
xhr.open('get', 'http://localhost:8080/bookmark', false)
xhr.send()
const raw = xhr.responseText
const result = JSON.parse(raw)
document.write('Your page is ')
document.write(result.page)
</script>
<meta charset='utf-8'/>
</head>
<body>
</body>
</html>
- Make sure the server is running.
- Open the page in an internet browser.
- It shouldn’t be functional.
- If you open the console, you would see an error:
Cross-Origin Request Blocked:
A sad web browser
The Same Origin Policy disallows reading the remote resource at http://localhost:8080/bookmark.
(Reason: CORS header ‘Access-Control-Allow-Origin’ missing).
“What the hell is CORS, sir? You promised you’ll help me. Fuck you!” you’re saying. Well, I’ve been there too once. Just know that CORS is a feature of browsers to stop web developers from stealing bandwidth from each other unless given permission.
Our website doesn’t work because the frontend and backend are on different domains. There are two fixes for that. Serve the frontend through the backend, or allow foreign connections to the backend. I think the latter is better.
- run
npm install cors --save
- Open the “server.js” file and add:
const cors = require('cors')
app.use(cors())
- Restart the server.
- Refresh the page.
- Double BOOM! 🙌🙌

Submit data
If your brain isn’t blown yet, then you still have time to prepare a towel. Come on.
Backend
To submit data, it is good practice to use the POST, PUT, and PATCH verbs. Today we will use POST, which is technically wrong for our use case according to RESTful guidelines. But I don’t want to spend time explaining that and your teacher probably doesn’t know anyway.
We add an endpoint. When you send a POST request it will go (see code a bit below.)
No questions? Good. “But… but how can I test it?” you’re saying. Damn! That’s a good question. Browsers don’t make it easy, so the answer is with Postman. Postman is a program for making other types of internet requests.
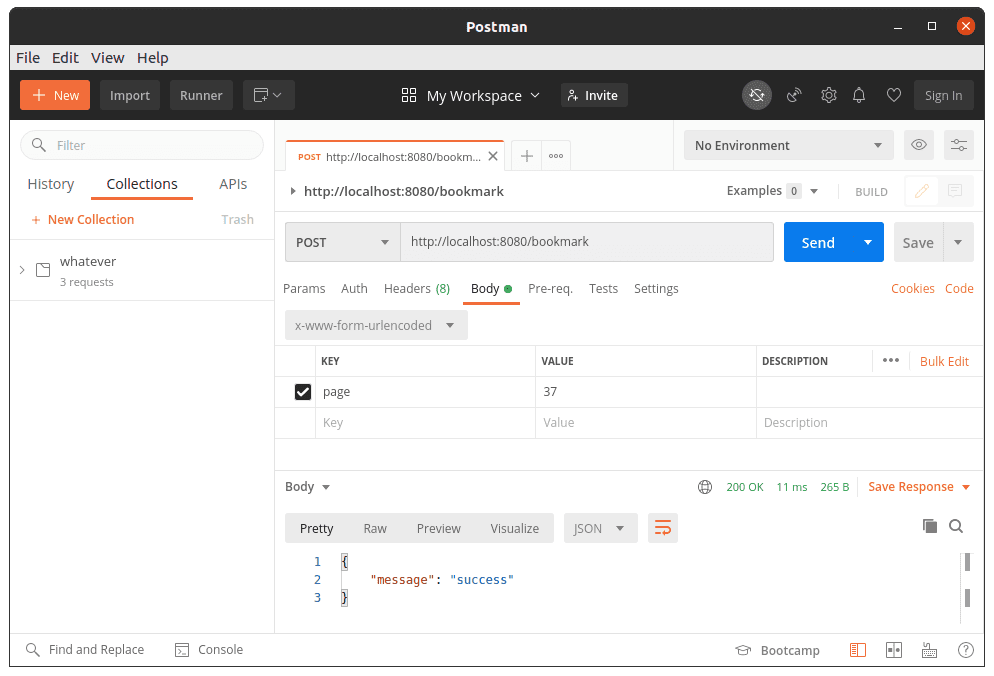
I am tired. And you? Regardless, triple BOOOM!! 💥🤯💀
This is the final code for the backend:
const express = require('express')
const cors = require('cors')
const app = express()
app.use(cors())
app.use(express.json())
app.use(express.urlencoded({ extended: true }))
let page = 0
app.post('/bookmark', (req, res) => {
const newPage = req.body.page
if (newPage === undefined) {
res.status(400).json({ message: 'page was undefined' })
return
}
page = newPage
res.status(200).json({ message: 'success' })
})
app.get('/bookmark', (req, res) => {
res.json({ page: page })
})
app.listen(8080, () => {
console.log('Server is running on http://localhost:8080')
})
Frontend
We very close. A little bit more. To the frontend! Come on!
We simply add a form (this is the final version of the frontend.)
<html>
<head>
<script>
const xhr = new XMLHttpRequest()
xhr.open('get', 'http://localhost:8080/bookmark', false)
xhr.send()
const raw = xhr.responseText
const result = JSON.parse(raw)
document.write('Your page is ')
document.write(result.page)
</script>
<meta charset='utf-8'/>
</head>
<body>
<form action="http://localhost:8080/bookmark" method="POST">
<div>
<label for="page">New page</label>
<input name="page" id="page">
</div>
<div>
<button>Yes</button>
</div>
</form>
</body>
</html>
Quad BOOMMMM!!!! 🥳🎊🎂🎁
Demo
It works!
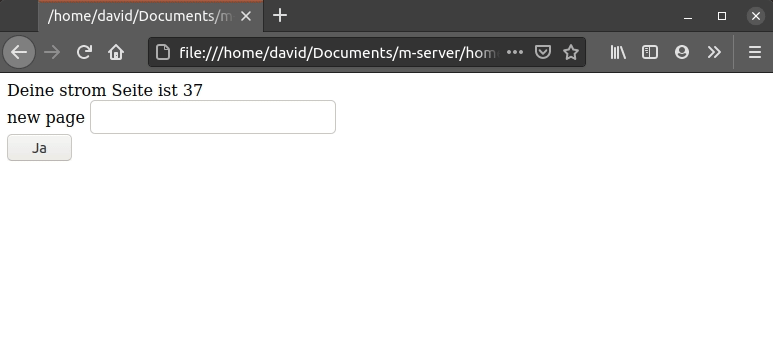
… kind of 😉
Summary and tips
We did it! You succeeded in making an app that saves data in the cloud. Congratulations!
“How does that function? How does it remember the number between updates?” you’re asking.
Ouf, you ask big questions. But I will try to explain: the server is always running until you stop it or it crashes. And the variable that tracks our page is kept alive because the variable was defined in the global scope.
If you require a database, I recommend MongoDB.
If you are required to validate input, click the link.
If your project is large and will last a year and must have a nice look, I recommend learning a frontend framework like Angular, React, or Vue.
If you continue with web development in general, I recommend learning TypeScript.
I hope you enjoyed the article.